If your Angular app needs to let users have multiple language options, the best way to do it is with a translation library. This is referred to as internationalization, or i18n for short.
Fun fact: i18n seems like a weird name – but it’s just shorthand. The word ‘internationalization’ has 20 letters… i<18 letters>n … i18n! You might see l10n mentioned sometimes too – that one is shorthand for ‘localization’.
Maybe you want to support two languages – say English and Swedish. Using the angular-translate library, this is a piece of cake. Here’s how to do it.
Install the package
Assuming you’re using Bower, install it this way:
bower install angular-translate
If you’re not using a package manager, you can grab the library from here.
But furthermore, if you’re not using a package manager, you really should be! Learn more about Bower and set that up as soon as you can. It’ll make your life a lot easier.
Include the script in your HTML file
Change the path if you need to (your angular-translate might be installed somewhere else).
<script src="bower_components/angular-translate/angular-translate.js"></script>
Make your app require the library as a dependency
var app = angular.module('theApp', ['pascalprecht.translate']);
Add the translations
var app = angular.module('theApp', ['pascalprecht.translate']);
app.config(function($translateProvider) {
$translateProvider.translations('en', {
TITLE: 'Welcome!',
MESSAGE: 'This app supports your lanaguage!'
});
$translateProvider.preferredLanguage('en');
});
Here, we’re injecting $translateProvider
into the app’s config function. This is a service that angular-translate makes available, and it is what we use to specify the different languages the app will support.
The other imporant part is calling preferredLanguage
. angular-translate will not choose a language for you, even if there is only one option. You have to specify it.
Translate some stuff!
In your views, instead of using plain text, we’re going to put in the corresponding translation keys.
Before
<body ng-app="theApp">
<h1>Welcome!</h1>
<p>This app supports your language!</p>
</body>
After
<body ng-app="theApp">
<h1>{{ 'TITLE' | translate }}</h1>
<p>{{ 'MESSAGE' | translate }}</p>
</body>
Be careful that you remember to put the keys in quotes, or it won’t work.
Now that it’s working, add more languages
In order to add other languages to the app, all we need to do is call the translations
function again. Those calls can be chained, as in this example:
var app = angular.module('theApp', ['pascalprecht.translate']);
app.config(function($translateProvider) {
$translateProvider.translations('en', {
TITLE: 'Welcome!',
MESSAGE: 'This app supports your lanaguage!'
})
.translations('sv', {
TITLE: 'Välkommen!',
MESSAGE: 'Denna app stöder ditt språk!'
});
$translateProvider.preferredLanguage('en');
});
Let your user choose the language
It’s not all that helpful to have multiple languages if your user can’t choose the one they want. This is easy to do with the $translate.use()
function. At this point we need to add a controller to allow selecting the language.
var app = angular.module('theApp', ['pascalprecht.translate']);
app.config(function($translateProvider) {
$translateProvider.translations('en', {
TITLE: 'Welcome!',
MESSAGE: 'This app supports your lanaguage!',
en: 'English',
sv: 'Svenska'
})
.translations('sv', {
TITLE: 'Välkommen!',
MESSAGE: 'Denna app stöder ditt språk!',
en: 'English',
sv: 'Svenska'
});
$translateProvider.preferredLanguage('en');
});
app.controller('HomeCtrl', function($translate) {
var ctrl = this;
ctrl.language = 'en';
ctrl.languages = ['en', 'sv'];
ctrl.updateLanguage = function() {
$translate.use(ctrl.language);
};
});
The view has a select
dropdown which allows choosing English or Svenska.
<body ng-app="theApp" ng-controller="HomeCtrl as ctrl">
<h1>TITLE</h1>
<p>MESSAGE</p>
<select ng-options="language | translate for language in ctrl.languages" ng-model="ctrl.language" ng-change="ctrl.updateLanguage()"></select>
</body>
See it all working in the Plunker here!
That’s it!
Now you know how to get up and running with angular-translate! Not too hard, right?
Try recreating it from scratch in your own Plunker to get some practice. The best way to learn this stuff is to practice it on your own. Don’t just copy and paste from tutorials!
The library has a lot more flexibility, too. Here are a couple other things to look into once you need them:
- Use the
translate
attribute to give translation keys without the braces{{ }}
syntax. - Extract your translations to separate JSON files and load them using
angular-translate-loader-url
. - Use the
translate
filter in services and controllers to translate things outside of the view.
Now get out there and start applying sweet i18n and l10n to your apps!
Learning React can be a struggle — so many libraries and tools!
My advice? Ignore all of them :)
For a step-by-step approach, check out my Pure React workshop.
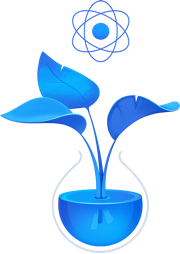
Learn to think in React
- 90+ screencast lessons
- Full transcripts and closed captions
- All the code from the lessons
- Developer interviews
Dave Ceddia’s Pure React is a work of enormous clarity and depth. Hats off. I'm a React trainer in London and would thoroughly recommend this to all front end devs wanting to upskill or consolidate.