Need to run a series of HTTP calls, and wait for them all to complete? Use $q.all
.
This wonβt work:
for(var i = 0; i < 5; i++) {
$http.get('/data' + i);
}
// At this point, all the requests will have fired...
// But probabaly, none of them have finished
Do this instead:
var promises = [];
for(var i = 0; i < 5; i++) {
var promise = $http.get('/data' + i);
promises.push(promise);
}
$q.all(promises).then(doSomethingAfterAllRequests);
Run the promsies in order (not in parallel)
When you queue up promises like above, they all start at the same time. But what if you want them to run in the order you called them?
You can build up a chain of promises:
var chain = $q.when();
for(var i = 0; i < 5; i++) {
chain = chain.then(function() {
return $http.get('/data' + i);
});
}
// This is the same as:
chain = $q.when();
chain.then(function() {
return $http.get('/data1');
}).then(function() {
return $http.get('/data2');
}).then(function() {
return $http.get('/data3');
}).then(function() {
return $http.get('/data4');
}).then(function() {
return $http.get('/data5');
});
$q.when
is used to kick off the chain with a resolved promise.
Learning React can be a struggle — so many libraries and tools!
My advice? Ignore all of them :)
For a step-by-step approach, check out my Pure React workshop.
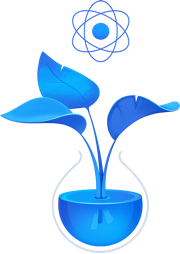
Learn to think in React
- 90+ screencast lessons
- Full transcripts and closed captions
- All the code from the lessons
- Developer interviews
Dave Ceddiaβs Pure React is a work of enormous clarity and depth. Hats off. I'm a React trainer in London and would thoroughly recommend this to all front end devs wanting to upskill or consolidate.