Pure Redux
With a patchwork of boilerplate stitched together from 18 convoluted tutorials...
…a whole day gone, and Redux still doesn’t make sense.
After hours of struggling, you’re about to rip your hair out trying to understand how to use Redux with React.
First there’s all the jargon – actions, reducers, middleware, thunks, mapStateToProps…
Having to look up every third word in the docs, it feels like learning a new language.
And beyond that, how do you actually use it to make React apps?
setState was so easy…
Plain React state is straightforward.
You call setState, the component re-renders, and… that’s it. It’s scoped to one component. No hopping around between files. Easy.
Redux is… not
Redux is a different beast altogether.
You have to set up a store. You have to write a reducer. Maybe add some middleware (don’t forget to compose it!). Wrap your app in a Provider. Connect certain components (but not all of them!). Dispatch “actions,” which don’t appear to do anything…
Oh, you just wanted to make an HTTP call? You need to install redux-thunk for that. Or redux-saga. It’s really up to you. Have fun deciding!
It’s like a choose-your-own-adventure Rube Goldberg machine.
The way most tutorials present it, every little addition requires a frantic dance between 4 different files.
And Redux is massively popular. By some estimates, 50-60% of React apps are using this thing. How did they all figure it out?
Most tutorials just add to the confusion
There are nearly infinite React-Redux tutorials out there, but most of them merely tell you what to type without saying why.
At the end, you’re left with a tangled mess of boilerplate that you only barely understand. And the knowledge, like a mirage, vanishes as soon as you look away. Then you’re back to where you started.
After all the tutorials, you’re still unable to build real apps with Redux and React.
How many more hours will it take to escape the cycle?
The biggest "aha" has been the reminder that there's no magic in Redux. I appreciate the well thought out exercises and the verbal and written encouragement to complete them. They're what pull it together.
Large examples are overwhelming to sift through
You know the ones. “I built a whole clone of Yelp/Slack/Spotify/Instagram, check out the Github!”
The theory is, if you analyze a “real world” app, then you’ll understand how to build your own.
The problem is, it’s like learning to paint by staring at the Mona Lisa.
Sure, the painting is great… and if you have a background in fine art, you might even learn something new. But if you’re still learning how to hold a paintbrush? Analyzing a finished product – even the best one in existence – isn’t gonna help.
Your teaching method is great - small pieces and practice a lot. I pretty much knew this intuitively before but was not doing it. You put it into practice very well.
Break the Cycle
What if you could finally build React + Redux apps on your own?
Arrange the pieces in your head – orderly and straightforward – and then simply snap the code together like Legos.
You could enjoy building a great product and contribute meaningfully to your team.
By starting with something you understand – plain React – you can build upon that foundation to learn Redux bit-by-bit. One concept at a time.
An easier way to learn Redux.
You can be productive with Redux and React, and this video course will help you do it.
You’ll get hands-on practice by building an app in React, and then refactoring it to use Redux. That’ll give you the basics.
Then we’ll walk through building a larger app, using all the skills you learned and practiced (plus a few new ones). One step at a time, we’ll assemble an app from the ground up.
Exercises help the concepts stick
There are exercises built in, to help you remember what you learn. This isn’t a “copy, paste, watch it run” tutorial.
And, as a fellow person who likes to know why things work the way they do, I’ve taken time to explain the reasons behind the concepts.
Comprehensive videos, easily digestible
Over the course of 43 video lessons (about 4.5 hours), you’ll learn all the fundamental concepts, plus how to actually use them in a React app.
All of the videos are under 12 minutes, and the average length is about 5 minutes. Get in, learn something, get out.
An hour spent fumbling around is an hour wasted, and your time is valuable. I’ve edited the videos to make them information-dense, while also keeping a good pace. (you’ll see a preview below)
The course is broken up into small chunks (one thing at a time!) and each concept is presented in a simple, unintimidating way.
Dave Ceddia is one of my favorite tutorial authors. He has an incredible gift for explaining complex concepts in clear and simple terms that make it easy for beginners to understand.
I'm very happy to recommend Dave's new "Pure Redux" course. It covers everything from core Redux concepts, to useful tools that will make working with Redux easier, to testing and other real world examples.
If you're looking to learn Redux, Dave's "Pure Redux" is an excellent choice!
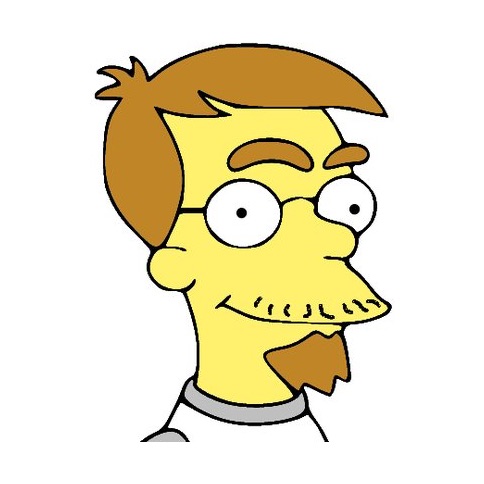
Module 1: Fundamentals of Redux
In Module 1, you'll learn how Redux works, and how it connects to React.
All those confusing terms you've seen? Reducers, action creators, mapStateToProps, mapDispatchToProps, connect... by the end of these lessons, you'll understand all of them, why they exist, and how they fit together.
The Basics
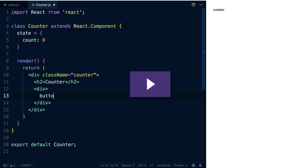
What is Redux? What's it for?
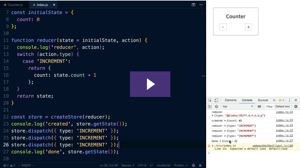
Start with a Plain React App
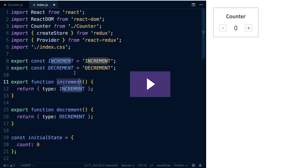
Add Redux to the React App
Core Concepts
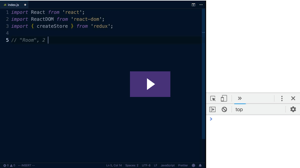
Actions
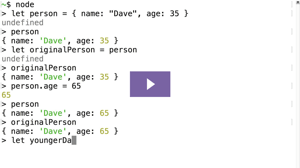
Reducers
Immutability in Practice
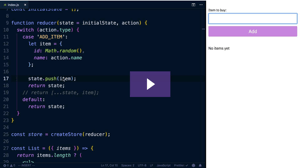
What is Immutability?
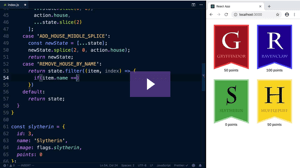
Why Immutability Matters to Redux
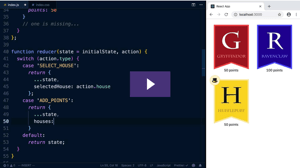
How to Immutably Update Arrays
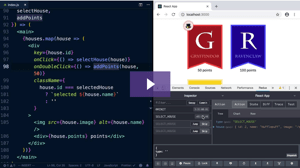
How to Immutably Update Objects
Useful Extras
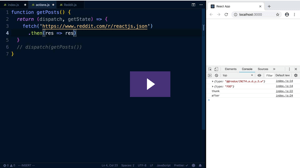
Easier debugging with Redux DevTools
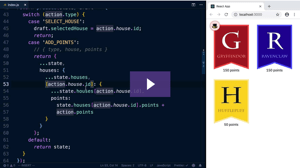
Make API calls with redux-thunk
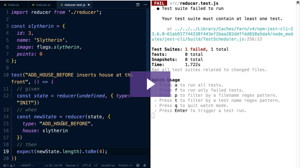
Easy immutability with Immer
Testing
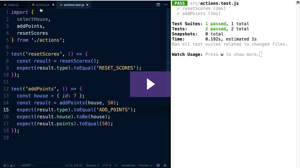
How to Test a Reducer
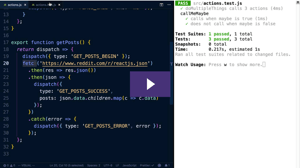
How to Test Plain Action Creators
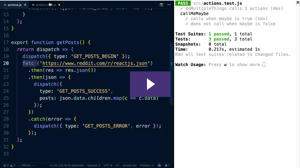
How to Test Async (thunk) Actions
Here’s a preview from Module 1, where we add Redux to a React App:
An early version of this video is up on YouTube, and has received comments like this:
I can't believe I got to understand Redux in 14 minutes after a whole day of not grasping how tf it works. Premium content.
As you know, YouTube is known for its positive and insightful comments 😂 And this video is the only the 3rd of 43 in the course :)
Module 2: Build the app
In Module 2, we'll build a whole app from scratch using what you've learned, and pick up some new skills along the way.
We'll start from nothing, set up a local API server, and build an app that includes routing, authentication, hiding features based on the user's role, and lots more.
This is the app we'll build, a courseware with Courses and Lessons, where admins can create courses and users can "buy" them:
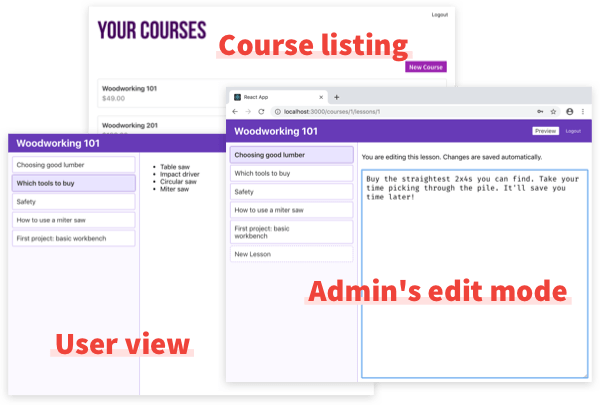
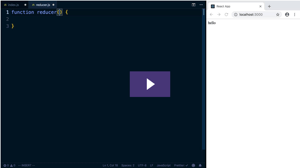
Create the OfCourse App
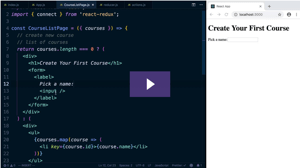
Use a form to get data into Redux
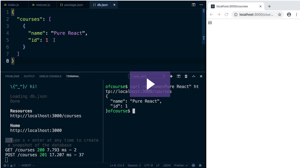
Set up an API server
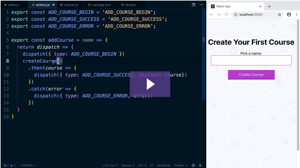
POST new records with redux-thunk
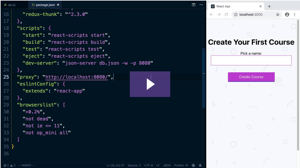
Proxy requests to the API server
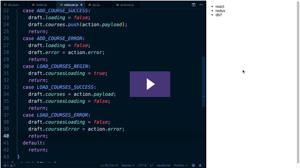
Fetch the list of courses
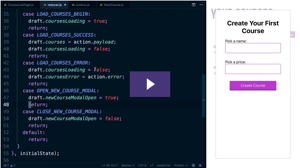
Add a "New Course" Button
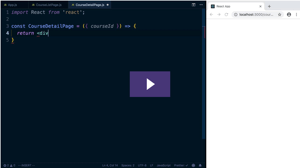
Add Routing and a CourseDetailPage
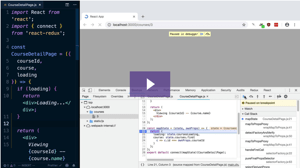
Fetching courses from the server
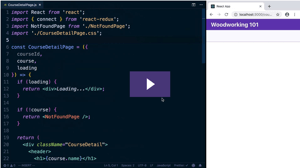
Style the CourseDetailPage
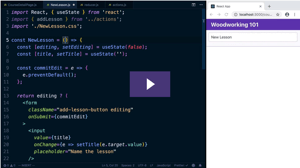
Add Lessons to a Course
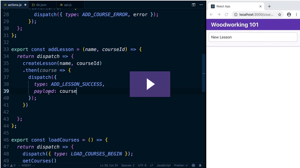
Save Lessons to the Server
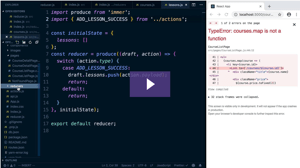
Split the Reducers and Use combineReducer
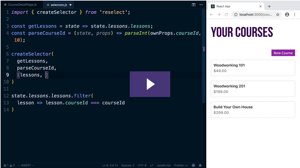
Implementing Selectors with reselect
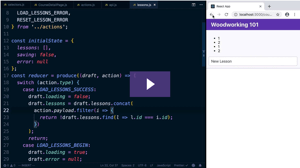
Fetch Lessons when route changes
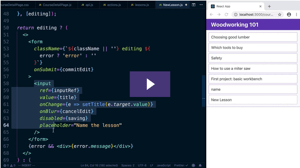
Edit Lesson Titles and Persist Them
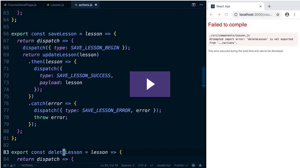
Delete Lessons from a Course
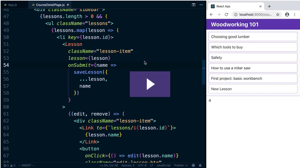
Create LessonPage and link the lessons
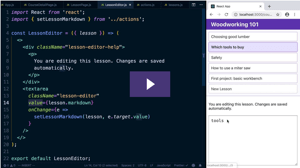
Edit lesson contents as Markdown
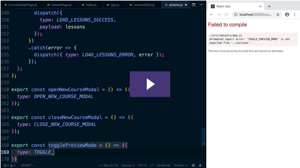
Preview the lesson markdown as HTML
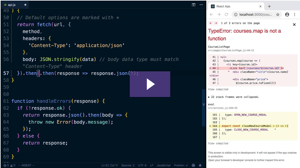
Fix the Error Handling in api.js
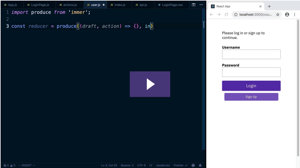
Create LoginPage, signup and login
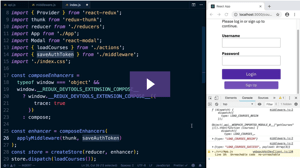
Save the JWT Token with Middleware
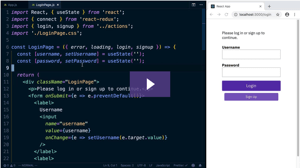
Redirect after login
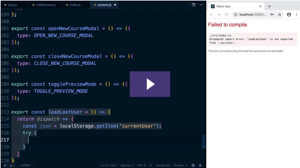
Save the user in localStorage
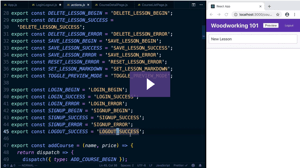
Logging out, and the LoginLogout button
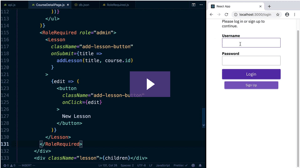
Hiding components based on role
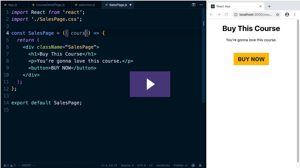
Let users buy courses at the SalesPage
Here’s a sample from Module 2, where we set up the LoginPage, signup, and login:
Redux isn’t always the right choice
Redux shines in larger apps where you have a lot of interconnected state. But it’s not perfect for every app, especially the smaller ones.
In those cases, the Context API might be perfect. It’s built right into React, and serves as a nice lightweight state management solution.
When you know both Redux and Context, you can pick the best solution for the problem at hand, like a pro.
Learn the Context API + Redux in one bundle
In Complete package, I’m including my course on React Context for State Management as a bonus.
Over the course of 11 video lessons (about 35 minutes), you’ll learn how to use React’s Context API as a simple way to manage state.
Usually available only with an egghead subscription ($250/year), I’m able to provide it to you in this bundle because egghead is awesome (and lets instructors own their content)!
In the Context course we’ll build a mail client. You’ll learn how to handle techniques like login and notifications, as well as performance and testing.
Your Context course helped me out with the basics recently when I was stuck. This is just as important to me as Redux.
React Context for State Management
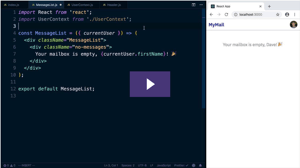
Use Context to avoid prop drilling
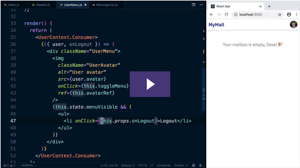
Allow Children to Update Parents with a callback
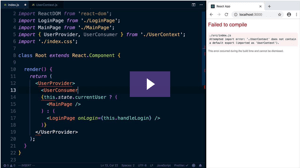
Hide the implementation details of a Provider
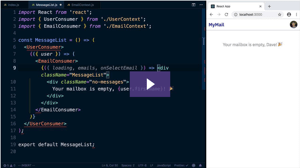
Use Multiple Providers in the Same App
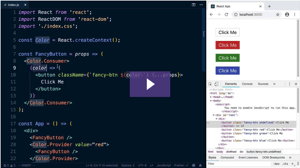
Use a Consumer Outside the Matching Provider
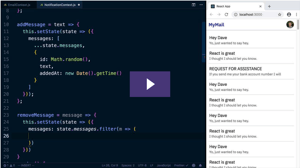
Use Context to Display Notifications
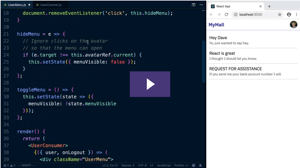
Use contextType to access Context without a Consumer
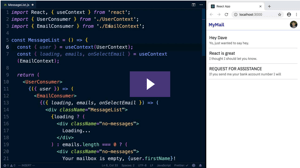
Use the useContext Hook to access Context in Function Components
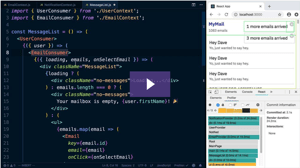
Performantly Render a Large List with React Context
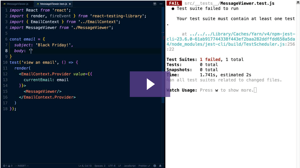
Test a Component That Uses a Consumer
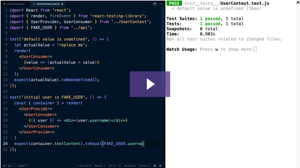
Test a Component That Uses a Provider
How much longer could it take?
If you’ve already invested hours – or even days – trying to learn React and Redux, how much more time will you need to spend before it “clicks”?
And how much is an hour of your time worth?
If one 12-minute video can save you a day of struggle, how far could you get with an entire course?
Buy Pure Redux
- 2 modules + Context course
- Fundamentals of Redux
- Build an app start-to-finish
- Selectors, authentication, routing
- 43 videos
- 13 exercises
- Code for every lesson
- Bonus! React Context for State Management course
- Learn to manage state two ways:
with and without Redux - Use Context Consumer & Provider to pass data easily
- Learn contextType and the useContext hook
- Learn to test Context-based components
- 5 hours of focused lessons
- 1 module
- Fundamentals of Redux
- 15 videos
- 11 exercises
- Code for every lesson
- 1.5 hours of focused lessons
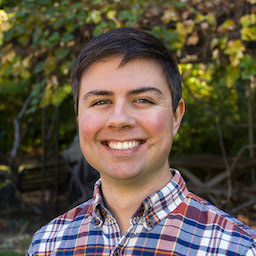
Hi! I'm Dave Ceddia 👋
I’ve been writing software professionally for 10+ years, and React is my favorite technology by far. I'm a React educator, blogger, author of Pure React, and instructor at egghead.io. I hope you enjoy the course!
What other developers are saying
Dave Ceddia is one of my favorite tutorial authors. He has an incredible gift for explaining complex concepts in clear and simple terms that make it easy for beginners to understand.
I've read thousands of articles on React and Redux, and many of the top posts I frequently link to as references are from Dave. Whenever I see a new post from Dave, I know it will be excellent and informative.
On that note, I'm very happy to recommend Dave's new "Pure Redux" course. Dave has created a screencast-based series with the same high quality, attention to detail, and clear explanations as his blog posts. It covers everything from core Redux concepts, to useful tools that will make working with Redux easier, to testing and other real world examples.
If you're looking to learn Redux, Dave's "Pure Redux" is an excellent choice!
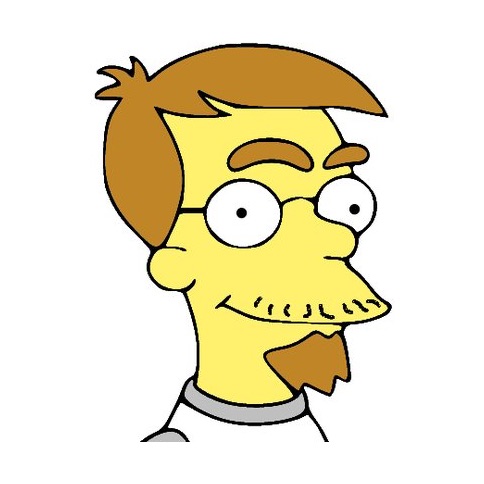
I really appreciate that you've created the course. It helped me to better understand the flow of a react-redux app and it's also a good reference when I forget something.
I bought it mainly because the course has a testing section and I didn't find that anywhere else and it's also full of examples and sample applications. I like the structure of the course and videos too.
The biggest "aha" has been the reminder that there's no magic in Redux. I appreciate the well thought out exercises and the verbal and written encouragement to complete them. They're what pull it together.
Your Context course helped me out with the basics recently when I was stuck. This is just as important to me as Redux.
Your teaching method is great - small pieces and practice a lot. I pretty much knew this intuitively before but was not doing it. You put it into practice very well.
Dumping everything into one file actually helped me remove some of the magical thinking I had with React/Redux. I have my pattern of separating everything out, and I'm going to stick with that, but it helped me think of all of the setup as "just code" that you can manipulate at will.
I needed an intro into Redux and after having bought your Pure React book I figured the Redux course would be a logical next step. It's short and to the point, doesn't introduce you to a lot of new concepts at once.
I like your approach to learning as not only being a mental thing but also a physical process. Without typing the actual code, the stuff you think you understand by reading does not last very long. True and very recognizable.
FAQ
Q: How much do I need to know about React to take this course?
The Pure Redux course is, as the name suggests, all about Redux. It doesn’t teach React.
Redux builds upon ideas and concepts from React – concepts you’ll miss if you try to learn both simultaneously.
So I suggest learning React before learning Redux. Learn one at a time, and it will (paradoxically) make you productive faster than learning simultaneously.
To make it easier to learn both, I put together a “master package” with my book Pure React plus everything above. In one package you’ll get Pure React, Pure Redux, and React Context for State Management. Everything you need to build awesome React apps in one place, taught in a consistent way.
Buy the React + Redux bundle ($149)
Q: What’s the format of the course?
The course is made up of video lessons and text-based exercises that you can work through at your own pace.
It’s hosted inside the Podia platform, which works similarly to most other courseware out there. When you purchase, you’ll create an account that will grant you access to the course. Take a look at the screenshot above to see what the interface looks like.
Q: So… it’s not a book?
Nope, it’s a video course. Yes, I know my last product was a book. I thought I’d mix it up this time.
Q: How long will the course be available once I join?
Forever! At least until I die and stop paying my hosting bills, or until the heat death of the universe.
You can go at your own pace.
Q: What’s the refund policy?
If you’re not satisfied with the course, just send me an email and I will refund your money.